## Deploying Your App
What needs to be deployed...
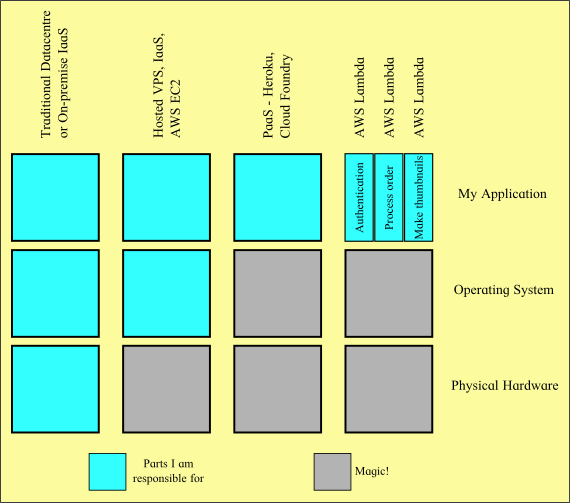
## What is AWS Lambda?
"_AWS Lambda is a compute service that runs your code in response to events and automatically manages the compute resources for you..._"
* deploy __functions__ not applications
* the code is executed in __response to events__ on other AWS services, such as S3, SNS, DynamoDB etc..
* or executed by the Amazon __API Gateway__ (⇦ choose this one!)
* only supports Java and Node.js ☹
## Adding some Python
We can make it work by getting a node.js script to execute the Python script!
* [WillyG - Python on AWS Lambda](http://willyg302.github.io/blog/posts/2015-03-29-python-on-aws-lambda/)
* [Tim Wagner - Using Python in an AWS Lambda Function](https://aws.amazon.com/blogs/compute/using-python-in-an-aws-lambda-function/)
* [Eric Hammond provides a wrapper function](https://github.com/alestic/lambda-function-wrapper)
## 1.1 - _Hello World_ in Python
* accept a JSON object from the wrapper, write JSON to stdout
* use virtualenv to bundle in python and extra libraries
## 2.1 - Lambda Wrapper Script
* This script runs our Python script
* Communication is via "stringified" JSON
var spawn = require('child_process').spawn;
exports.handler = function(event, context) {
var response = {};
var error = null;
var child = spawn('venv/bin/python', [
'lambda-function.py',
JSON.stringify(event, null, 2)
]);
child.stdout.on('data', function (data) {
console.log("stdout:\n"+data);
response = JSON.parse(data);
});
child.stderr.on('data', function (data) {
console.log("stderr:\n"+data);
error = { error: true, message: data.toString('utf8') };
});
child.on('close', function (code) {
if (error !== null ) { context.fail(error); }
else {context.succeed(response); }
});
};
## 2.1 - Lambda Function
* upload a ZIP of the directory to S3 bucket
* ```zip -r /tmp/hello-lambda.zip *```
* upload... this takes a while to upload 39Mb of Python
* make an IAM role for executing the function
* create the Lambda function
## 2.3 - AWS Lambda Console
Once it is running, use the console to check logs and number of calls.
[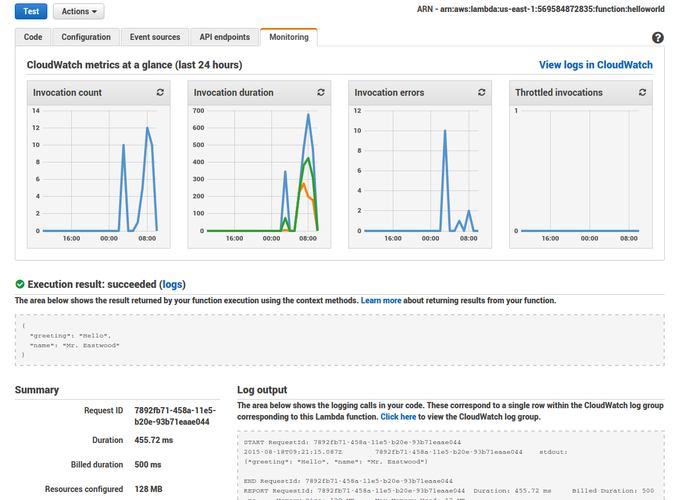](talk/aws-lambda-console.png)
## 5.1 - API Gateway
Configure a new API on AWS, with a resource that executes the Lambda function.
[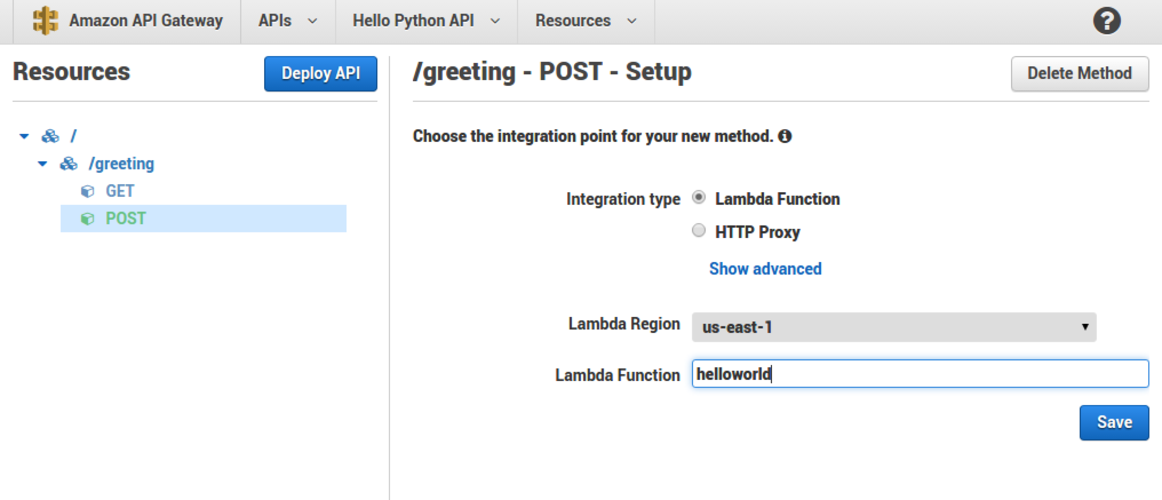](talk/aws-gateway-1.png)
## 5.2 - Say “Hello” to my little friend
$ curl -X GET https://hostname/test/greeting
{"name":"Mr. Eastwood","greeting":"Hello"}
$ curl -X POST -d '{"name": "Clint"}' https://hostname/test/greeting
{"name":"Clint","greeting":"Hello"}
## 5.3 - Again... with Pictures
[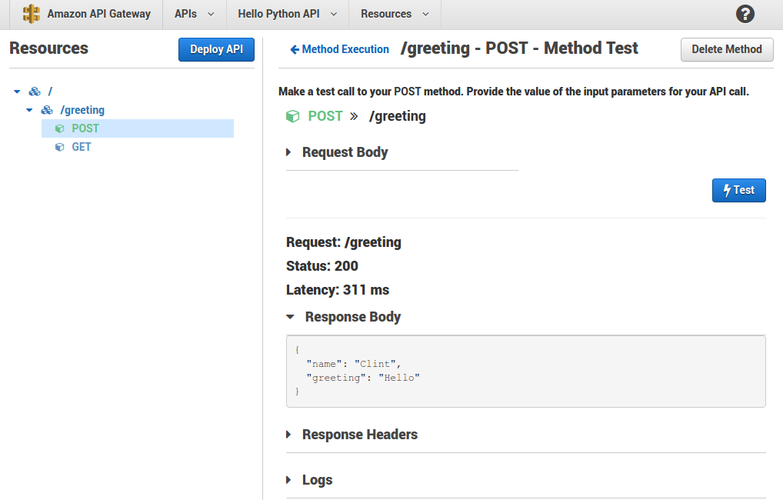](talk/aws-gateway-4.png)
## Conclusions
* it's __cheap__
* FREE - first million requests per month
* then $0.20 per 1 million requests
* minimal deployment hassle
* no EC2 instance, no server maintenance
* great for a __micro-service__
* simple endpoint
* reacting to an event
* terrible for a Django app